Rest API
Source code for this can be found here.
Overview
In a scenario, we have a basic medical clinic where people can come to have check ups, request copies of their medical records, or if they're feeling sick they can get treatment. There is a desk at the clinic with a medical secretary and shelf full of medical records. Before getting help from the staff you have to fill out the patient check in paperwork, place it inside of envelope, fill out the envelope and hand it to the staff. As instructed, patient 1, fills out the paperwork, marks it as request info and hands it to the medical secretary. The staff then looks it up in the system and verifies the social security. She then checks the reasons for visit on the patient paperwork and sees that he just wants a copy of medical records. Because he is who he says he is she makes a copy and hands it over to him. Patient number 2 comes in and puts reason for visit as "Destroy all records" After this he hands it over to the receptionist.
Although the patient records are a match, he is not able to fulfill his request. Requesting the destruction of one's own medical records is okay, but not the destruction of the records of everybody is not legal. The receptionist tells him to get out so he does. From this example we see that the secretary acts as a buffer to the medical records. The patients have to identify themselves to the secretary, she verifies who they are, and looks at what they want to do. If they are who they say they are and allowed to do it she goes ahead and does it for them. The medical secretary represents the rest API, and the medical files represent backend database and the patients represent end users. The boxes on the envelope would represent common HTTP request methods. HTTP GET, PUT, POST,DELETE. Whenever a client communicates with web server it has to specify one of the http request methods. The clinic of patients represents the URL. The patient first, last, and social security, will be used as authentication and will be placed in the header section of API. Lastly reason for visit will be the body section of API calls. Its usually used to identify type of information that you're requesting.

This is an example of an actual API call a browser might send to the Rest API call. This is equivalent of our patient walking in , filling out that check in paperwork and handing it to the receptionist. When rest API receives API call it will inspect header to make sure credentials are correct. If credentials are correct then body is inspected. If user that was defined in the header is allowed to make the request that was described in body, the rest API will retrieve database and return it to client. After the browser receives raw data, it will do some magic with JavaScript and display a web page for user to look at.
In this project I will be coding this Rest API using NodeJS and Express. The API will have four functions:
- HTTP GET - Allow patients to request copy of medical records
- HTTP PUT - Allow patients to update their information
- HTTP POST - Allow patients to register as new patients
- HTTP DELETE - Allow patients to delete their medical records
To follow along what is needed is NodeJS, VSC to write Code in and Postman. Postman allows us to send API Requests to API and test things as we go along.
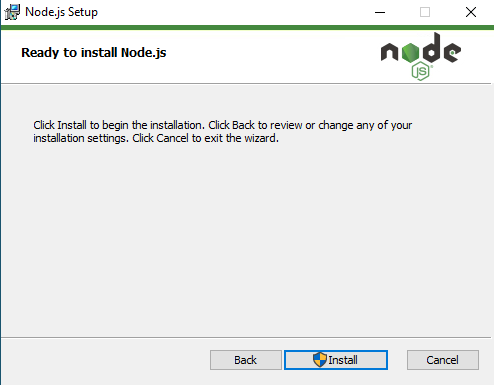
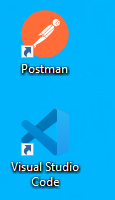
To start make a folder on your desktop called API to save our project files. Then open up VSCode and run as administrator. A module needs to be installed to require admin rights. Go to file > Open Folder where the API call was saved. Go to terminal to initialize project and install modules. "npm init" initializes project for you and accept all defaults. When its finished it will create package.json.
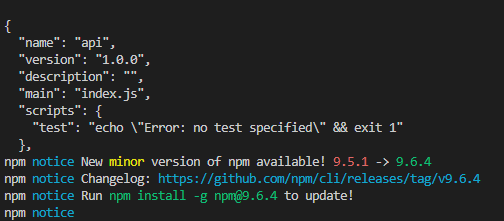
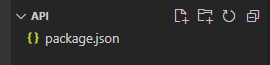

This file is used to track dependencies and modules. The module we will be using is Express which is used to let our API easily accept web requests. Npm install express in terminal. Npm install body-parser which is used to parse the body of API requests. The last thing to install is nodemon which is used to automatically restart your server when you save the file you're working on. This is done with npm nodemon -g , with -g being for global.

We are now ready to begin coding. All the source code for this project will be uploaded to my GitHub. Some notes:
Postman works similarly to a browser. You enter the webserver that you want to sent the request to.
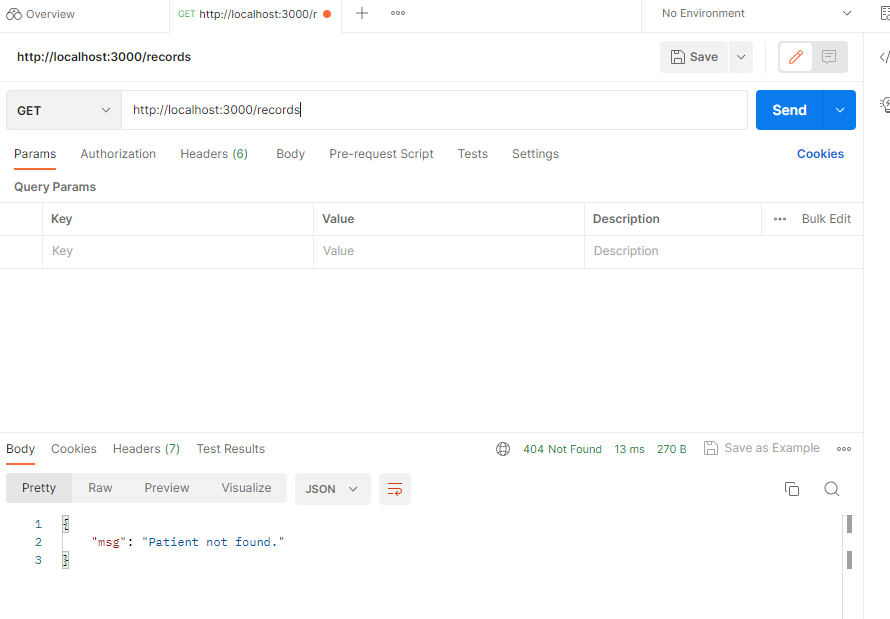
If you are the one implementing API, you can put any status that you want. Generally you want to send statuses that make sense. Normally there will be some type of backend database that holds patient records. Instead for this scenario, I will be using a hash table that holds our patient records inside program.
Functions using API call:
Get status of Lebron James:

I also added functionality for creating a new patient, updating the phone number,and deleting patient records.
Conclusion
This was our implementation of this medical record situation. A way to improve this would be:
- Expand patient database
- Different values in header
I plan to improve this in the future with additional functionality.