Linux Command Line
1 - Hello Bash Scripting
![kali ) -C —'Desktop/projects] /etc/shells # /etc/shells: valid login shells /bin/sh /bin/bash /usr/bin/bash /bin/rbash /usr/bin/rbash /bin/dash /usr/bin/dash /usr/bin/tmux /bin/zsh /usr/bin/zsh /usr/bin/zsh /usr/bin/pwsh / opt/microsoft/powersheII/7 /pwsh /usr/bin/screen](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e862672fec437e3f1b4e_EIICxrewlUCEykHpIevU1qRH9pN-Qvg5JNsNLRara87pIfbPo3NMy6uS7r8ChxaKHgEuSEGBlNdJHffV-dmAN8-TQPkC9yR67tSzZQxU4soAwHyHWstjfhpP9bu2io6Gueu--rgc-346B809qhcMKjY.png)
What this command does is shows all the available shells on system. Any can be used.
![kali)- which bash /usr/bin/bash —/Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e86941d4f4f0b8764cca_JXzTW-QwwBYw72VdPmnHyynmVlAlrZyGxdEYzDkLOP6uxWaLMaufXEXnMUB4a8LSrxY3gV9CEJ99aZXEz5j1XvEqHU66mYpKjLNS58hWNy41Gf63ccLlPyfzxSgr52ug9cujcnehC1_lollmFlwuTrY.png)
Shows path of bash. Add this to any script that is written
![kali —'Desktop/ projects] heUoscript. sh /usr/bin/bash echo "hello bash script"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e874ceb4275b40e92184_lrhSDkBjq7qKDN9HAsIe00q6EL3MA3gLpVSXhvmsVTYAwmxu4w5MWagrFxs5I6OMwFgoumSzCjqL2e2gasAkNs5OfATswDMjfhbxseqnc9uqFn43_TfD8HwRupLa6udO_HfTvMu9DligGYEun_6RYOE.png)
![(kali@ —'Desktop/ projects] drwxr-xr-x 2 drwxr-xr-x 4 11:18 total 12 -rw-r—r kali kali 1 kali kali 4096 kali 4096 kali 42 May May May 5 15:07 5 15:08 helloscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8783fadf2b4d107ef4e_YJr_Xa15edjRqTBcm_QoU4w8Idi3f0OfuFFbZI_TdNEzDy4gnrNSLxzzgv1lI_r6ivOR9cdvN5t_rVQW5nolNiQ5gFIMM6kZAR16zg7CjTyw2vy_fmHoCbpc70CIUDEyaFfHG20sNVELOuv40SnraZI.png)
In order to make script executable, have to add chmod +x to it.
![—'Desktop/projects] L-$ chmod heUoscript.sh (kali@ —'Desktop/projects] helloscript. sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8a4ff241583043716dc_JZbpgK9CDOSULkVAV7t2HMG8VpgAIglb9sIcGQSHFzh19cd48tYgZDp2wyeWacJxSty9pfNZo0eRFNndCdvBHdaLl3kTSDCJF8H6E1-ormeMOHCU3nlQ3iA3YY3jUmyb0YtPTNz9VjMJDLwu43HCdX8.png)
./ in front to run script
![(kali@ —'Desktop/projects] $ ./helloscript.sh hello bash script](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8aedec80530a20f9815_XKNTGHUx-PL604bkZgbTzpWLL966opOr0vPN952AteXnJInhxIJj8MZH-KiB3N8v90lknAaxevkOBBuvjznQiVLEdKSG5r6IkhW_1Y95qDDcYuhk3mPwUyf_P7yVKBdXfQ_ZRxRnV_k7KNnBsEofMo8.png)
2 - Redirect to file
Show how to capture output and then send it to a file.
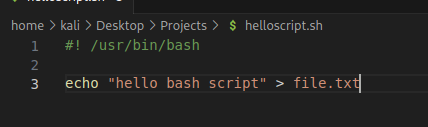
When ran, creates a file called file.txt
![(kali@ —'Desktop/projects] $ ./helloscript.sh (kali@ —'Desktop/projects] file . txt helloscript.sh —'Desktop/projects] file. txt hello bash script](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8c2bd58c06ddb550417_H8Dc5DDKTn3u-tNf_bzNaVIhKdS9zILP6VSjqsLFWdqvLa5u0g1m4NhyDhKxVVCL2gM-Ia10VaS452MDU7HsrdrN-piDr9lfOJfHWoJTpBOnFRuyFD-phULTo6sIzc11Am-dFICfsc2CUDEtC22rUrc.png)
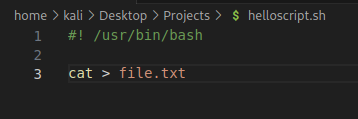
Script becomes an editor. Whatever is written when script is executed
![(kali@ —'Desktop/projects] —$ ./helloscript.sh lello, this is a shell overview, I am Jelani. Welcome](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d3f5fc6b1c0faaae54_L-9LF0CU93sPE54HQFglU4CfVrKdxjz3qkg69YOox63on6KOyTLj4HDZjbRNEgLO8tCf97M3kcyaRExscrYm3sqyMghewzVEtx_DxcNIeDX4_BiXaccASdY-pU5kfujE_JhHfiFqPonma6AWlHfyWdU.png)
In order to come out of it, you have to press Ctrl + D.
Output of one displays output of another. Essentially replaces text.
![kali@kali: —'Desktop/Projects File Actions Edit View Help (kali@ —'Desktop/projects] $ ./helloscript.sh hello bash script —'Desktop/projects] helloscript.sh /usr/bin/bash echo "hello bash script" (kali@ —'Desktop/projects] $ ./helloscript.sh (kali@ —/Desktop/projects] file . txt helloscript.sh —/Desktop/projects] file. txt hello bash script (kali@ —'Desktop/projects] $ ./helloscript.sh Hello, this is a shell overview, I am Jelani. (kali@ —/Desktop/projects] file.txt - Visual Studio Code File Edit Selection View Go Run Terminal Help Restricted Mode is intended for safe code browsing. Trust this window to enable all features. Man.ge Leam More $ helloscript.sh file.txt X home > kali > Desktop > Projects > file.txt Hello, this is a shell overview, Welcome @Restricted Mode @0A0 I am Jelani. Ln 1, coli Welcome Spaces: 4 I_JTF -8 LF Plain Text](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d3280d409cae9a4757_dVJqVlfC-NsVv_68fyKD-kw0sjwMr7A6dvQDbKbXmYwGO-Nb9oZgrqluvfePaL4ZTTkeESqsEI8syWVxbtQECox2R5MUBYuMrS3KBHqFB_wKNitraJXXKK8NgNPD7LR50ICJRtZjkXDZztSFmFCCFv8.png)
In order to append, put 2 >>
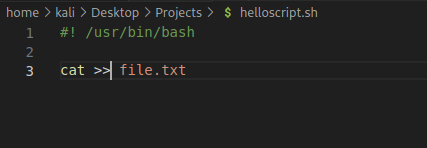
![(kali@ —'Desktop/projects] $ ./helloscript.sh This shows how to append to a file $ helloscript.sh file.txt home > kali > Desktop > Projects > file.txt 2 Hello, this is a shell overview, I am Jelani. . This shows how to append to a file Welcome](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d3fb95c232cad0ca8c_p2J-E2qEsEFbTTYSh-nO2YUUtD4WE9qNWM2M6gGl-P9_nErHxOpyShTKhw5zBhcrDhPliwZ2ZZCSSOY4iG1PbJHCOMQ-AirCcMTKLdabX_Rh_B2bDg_5zQmjuYqk6ZCE7qivQLXkMOdE8dZLj0IA2Wo.png)
3 - comments
Has no value except explaining code to programmer.

![(kali@ —'Desktop/projects] $ ./helloscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d339f6a9c478a2abf2_0F0mJja7PZentq7D98qEAKMdP-8ZopCJMwhTf2DZ_3_S53qCTo22lk6fKAPiVdz5N52VUsb3JDZcZgmV-qi98ZMP2JVrRIsvwWMfSM69QkzRXPhuO9Dim_byGG8TKnWXiP8mymly1s5COAKw5HqIUOU.png)
Doesn't show in execution of code. If there are 500 lines of code it does not make to out # on each individual line
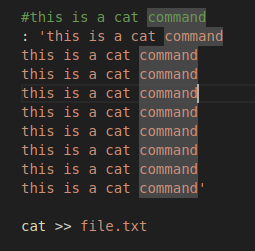
:'
' to multi line comment
Heredoc delimeter
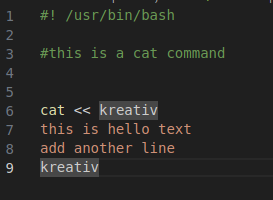

![(kali@ —'Desktop/projects] $ ./helloscript.sh this is hello text add another line](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d43fadf2b4d1097965_VkWPa1HtIB0BSi2byrKcIu96R7CYPGNAR3TofrFHWvjKpCnLTcsf9kGvRF5T19Ynf0ksU5cLwKwowNWvy6mscYUeH47L26nrSYE7Xhtq9QadOfqtGoumUDUv1ZOW5IsKkgzzYLnxtlEsaTQFHVzn_wU.png)
Similar to the variable like
4 - Conditional statements
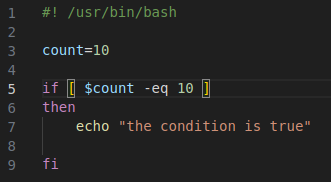

Inside if condition, checks that count equals 10. Since condition is true, execute the block.
![(kali@ —'Desktop/projects] $ ./helloscript.sh the condition is true](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d43e8b5f3f253f060f_A2byittmizlorMYDHhQ-xFAIJzhLK70sTzsK5sXfAccXvLRdgzeo9eR5wqDeywpj64lU0L3va0ppQHvWZo5ip05vZQpfxsuLEXVCE1kSpg0vlen_Z4LvtCztmBL6tESiJTT3VvwmuMXLaSvF1NRg5F8.png)
![2 3 4 5 6 7 8 9 /usr/bin/bash count—IO if $count -eq 5 j] t hen echo "the condition is true" fi](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d4ff2415830437de52_KTp8czKK7esq-TnhdrWknXPrqCR0Tf9tX8sIyJkzIEuBliri7oPJH1BplejqhneBUWqltROaYX6tdVMW9Z4P8IKO0cg9EN7ACmvrcC2qtCZmN8s7toKVl7OPK4Xvjm6f0eUZhML7PlLiz652QLxnKjE.png)
No output, since no else block and program doesn’t know what to do.
![2 3 4 5 6 7 8 9 1€ 11 12 /usr/bin/bash count—IO if [ $count -eq 5 ] t hen echo else echo fi "the condition is true" "the condition is false "l](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d43fadf2b4d10979a2_q-Tt3kvbBGys6CRiku90PJRy0IYG2ahmANA9ZaKCcRNxAqiLKuzaB9MZG6lhP5JgBowSp1t30QDKQaTdHb-1TQb197DD3NQQ2f9KCe7FMAiV_ZUMbgsilUs-lqqbN7moicJ4AvdWR_SuI-QuqZ_Nzws.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh the condition is false](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d41d4a687857afa592_J7r-e1hhZTKis17KolUFLGk-jfnH9tJZYT4yWwmkxk0lCedt7vImrmC_7fevJNUcU_95zO9tp5ZptnqbF1NvQ0Bu8DQXHd8WOepy5nk18jNobd7uV0juxhzcrtfSwUbC3PFHUgwMgjyQsK-MhjIv6M0.png)
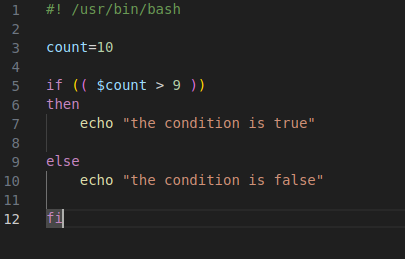

AND Operator
Checks if age is greater than 18 and less than 40
![2 3 4 5 6 7 8 9 12 13 /usr/bin/bash age—IO if [ "$age" -gt 18 ] t hen echo "age is correct" else echo fi " Sage " -It 4€ ] "age is not correct"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d496977b184c2588f9_RHcMs1JCpcYgDTR8ze2Np-tl6i2DIw6M-l8ebyeZRaqMYY1a0AaxLGuTAusCKCNwnK6u6f4DMNreOUYS31w7vd2HsYCKjdClwAkO-av1QHDrokwL72mkiLijbWNRnfmmpIWcmSwz47nLgBtCkGULZb8.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh age is not correct](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d496977b184c258902_12APHm5-n0vNc63lu7Qt7aGWB_vwBvfSULGoq_8sRRudfIbTzMHUvQYcsJfOkzZ9RRssR3QQikJKVkth7BTSBidVOZ9-tVqxmxwBRk7fis-Wouw_sMbXxKq5qSNE5uzx-a-9WvevYWG0PFn7uHDJ_Cs.png)
Both conditions have to be true
![home > kali > Desktop > Projects > /usr/bin/bash 2 3 4 5 6 7 8 9 10 11 12 13 14 age—IO if [ "$age" t hen echo else echo -It 18 S helloscript.sh " Sage " -gt 46 ] "age is correct" "age is not correct"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d46981a2bbda955c77_BRmmZBDLJ_kHPslZtlOw8VsHaUp_SnTrLBgfplK_zvspjBrjZBQW7AC1Fv8vbt0fQgeRr0aWJ8xdc6WAVyycf5b80q8pXjrcxnP7EGw1F_4nMOn_FG_goJymHHQ_TdvK35q2M6YFEf4tySiARMXNxRA.png)
One true condition, one false condition
![(kali@ —'Desktop/projects] $ ./helloscript.sh age is not correct](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d441d4f4f0b8778a0f_A0z5UXnwnFQQr7hXgzeW1TouJbeJNzr6vPKGg3n3qr0BEVbTbnqTftjVKBz1WPGJ6NDRaIQjaXKBTXtjIjR2EVPSLc1wXcrfV5nZaJEG0J-KIsQBEJgCPvrjzsJe2iK0pnimVz-8M0JYnfBN7X4dzaU.png)
![2 3 4 5 6 7 8 9 1€ 11 12 13 14 /usr/bin/bash age—IO if [ "$age" -It 18 -o "$age" t hen echo "age is correct" else echo "age is not correct" fi -gt 40 ]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d4711d826ac6c55f65_Z0xSLa-MSK9KLHDyjMuwX11kKRoDo8T8bGHT8j40aeOURoQOCQSP41l4iq4BWR-OdcCP-gD_4CEXlaxSbIW29qNVl5N9dOCAIZOfiQXukt8O5auW4jdM-JGquDZCu-SJ92Wd5NlBiYhwSg-gIVVUkVQ.png)
-o for OR operator . -o can also be substituted with ||
![(kali@ —'Desktop/projects] $ ./helloscript.sh age is correct](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d5e9537c2af204cc3c_oAqcp5KJCE5OB5Bq4YTOrY39PtlFt_mrRQ7gJB3-EvNv34wuIZFgm8xyg_xdLDRr6fFeRimxoT_KKtWK8Jgm2Fsu0XEU_Xskc_BA5IeTY9WtbBJ5yHOpD1K6c2QXAmGj-V3ssbG_U3T-FYwHEsamPXQ.png)
5 - Loops
While:
![2 3 4 5 6 7 8 9 /usr/bin/bash number—jl while [ $number -It 10 ] do echo "$number" ($number+,Ä ) ) done](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d43962f3dd04b1aa0a_-ErCQcM8yl7jcOOLlL8f-Bft8n2BSRstH4MVbZ7d4MiZVHR_s0ijM7fiQSNwiKy3h8NqBofKHD3ukbUVGmXHqvglq9ULm3kOS-HtG4jDx7Pifd1CR3GEhRTVJ_IeQFr3i4X-P0rJPrPKUACrwlbdAwk.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d4429a6e818365d4cc_h9N8C-9pfX3zyIqstrACK5Ffiv4JnRaYqGfP5m8yDq5LKi3yUR6yJw55O6_-1OVYmeQX-dfP6qDXBOIFwbugH8N-rcJKOhsuWXtP5ewb_yQ9eQp3e9u59BAlfa4uzCEw6wrI9OKYrn1lvkpjKB0oKB8.png)
For:
![(kali@ —'Desktop/projects] $ ./helloscript.sh S helloscript.sh X file.txt home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 /usr/bin/bash for i in O. .1$ do echo $i done](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d55c687ae1cd911a92_PfZdeH_2jHXmcEENUipQg3etrNuo0_SFBaqG4kOHWvq1awVYEpylXAi8q2nyJzM_hOxrIe7Qd63eRhXGOlK3hg3nVWVbY1oDQhWuBjh9kAqxKDHROL3ZAOF4X5kLTDRlqQu3GsE0wLIFCmQM5yelD_g.png)
![home > kali > Desktop > Projects > $ helloscript.sh (kali@ —'Desktop/projects] $ ./helloscript.sh (kali@ —/Desktop/projects] 2 3 4 5 /usr/bin/bash for ((i=€; i<5 ; i++)) do echo $i](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d51ee0df783dda32fe_wELkxqXVAIsa-Hq5vSAGqmACD9cUcN5_hQ-02nTXOSH36_a3zVxw3YcZTOk2nwPkDFPU4-wF4MNF_AAz70yymsxCChSIpXLCYv7r5_ivZ4GJQbume46KIMjTWvJxrqc5nZWVh2g1bhEO2CnFwotYqdQ.png)
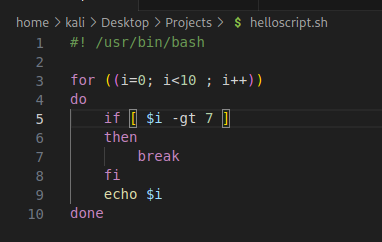
![(kali@ —'Desktop/projects] $ ./helloscript.sh (kali@ —/Desktop/projects] S helloscript.sh X file.txt home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 8 9 1€ /usr/bin/bash for ((i=€; i<10 ; i")) do if [ $i -gt 7 ] then break echo $i one](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d52bc54154aac13956_JrYnr2xQ5NXOI804aCiZm5XysjYr_YHf8jFCLwRD2a4370SKOgCvHVMR5oX1fzkCxiQQoE74JHLqlfb_8X70CUbDuMzvOKkPJGaFAVhD3XIL3XJDvSpFKdwzC6Nk1huvkMYGOpfQkBC8s6wKYUqmsDU.png)
6 - Script Input
Input represents $1, $2, $3
![home > kali > Desktop > Projects > $ helloscript.sh (kali@ —'Desktop/projects] $ ./helloscript.sh BMW Mercedes Toyota BMW Mercedes Toyota (kali@ —/Desktop/projects] 2 3 /usr/bin/bash echo $1 $2 $3](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d52c105913fdf6f68b_ktE1u5d0PmjKnI84tmQu06tgFkSVk9mHXfMXtxgIoT1mlTCH-bOvGgldicIMS3OUAPm70mn8rptvuBtTxSZ71BCSbqW5TaoASaFIAPmLHY0B_eRqgKDG6aKjDMwtF2AX2nw_whPThebcMtVTkR-WlAs.png)
Create an array with unknown number of inputs
![(kali@ —'Desktop/projects] $ ./helloscript.sh BMW Porsche Mercedes BMW Porsche Mercedes (kali@ —/Desktop/projects] 2 3 4 5 /usr/bin/bash echo](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d549132b6346fa1a3c_kTZ0SapE9mBRdMK9OnC2IrNBmDBXIt51eR-lQsYj8kFOkoqy4FAMCtYtsJFoy9EzuFy-M7FCwwlpvx9C4pVIwluF86QVmmTSIL-ad67DVuptXP4I3iHMnxZqQAh0QHl_YPyJ7t_uZl-NcSiob4rTMcc.png)

![home > kali > Desktop > Projects > $ helloscript.sh (kali@ —'Desktop/projects] $ ./helloscript.sh BMW Porsche Mercedes BMW Porsche Mercedes Hyundai Kia (kali@ —/Desktop/projects] Hyundai Kia 2 3 4 5 /usr/bin/bash echo $4](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d539f6a9c478a2acf6_5bbk_Cjlxo6VdsbvKPRWg3sG3EcKPWggMckRPxEpVUc_Z4qavW4GIchg-Lu4o0iGW99IVDeDdwPJnggyGupBBa7WViMSYWw9h83q0Dvx_-CmNz9Fc437Dkjrz-bHIV7_3Z1lK6tpLKVxFQzq8Bsfmh0.png)
Prints all the values that are taken in.
![(kali@ —'Desktop/projects] $ ./helloscript.sh BMW Porsche Mercedes BMW Porsche Mercedes Hyundai Kia (kali@ —/Desktop/projects] Hyundai Kia S helloscript.sh X file.txt home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 /usr/bin/bash echo $@ echo $#](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d5c171a922f2d2d54b_hryYAaGqvamPmDRDWt4ljGCg7KsJt5hFiECE8JdMwSVtnJ2sM7-lHgjv1YtRAVeHUZEp7oux2hHyP3dxa_V24f7hpdTshHOMXiuMDSGR09wAhUa3DFe6poe22d1jCK5xd42i8KiOvGCJNARFNoKWcI8.png)
Prints length of array as well
6 Script Input
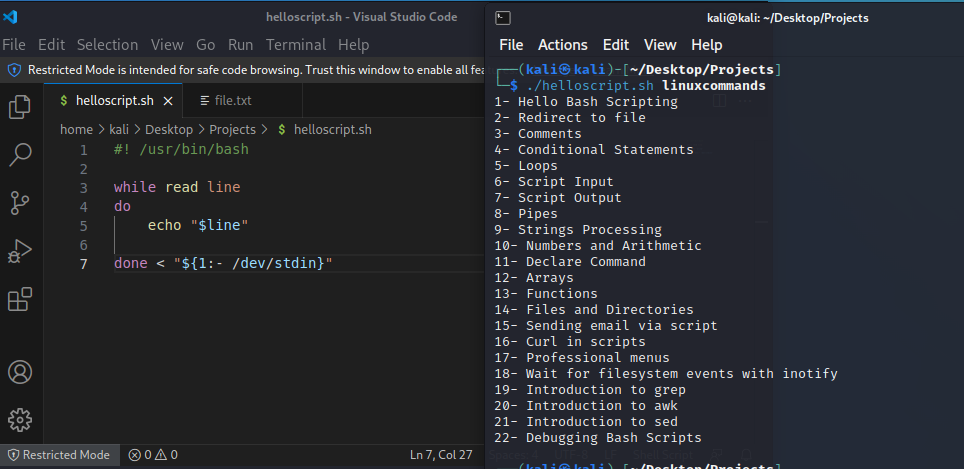
7 Script Output
![S helloscript.sh X file.txt home > kali > Desktop > Projects > 2 3 Is /usr/bin/bash -al l>filel.txt S helloscript.sh 2>file2. txt (kali@ "Desktop/projects] $ ./helloscript.sh (kali@ —'Desktop/projects] filel . txt file2 . txt file . txt helloscript.sh (kali@ —'Desktop/projects] Iinuxcommands](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d512cb93aaa98e9492_VHXS-eL_iIDT7n9x7X9ae8uyTL2JeM_-5M_nAuS3w3X39qp_whK2_aqyvKhrlNhnAeSMD3V2Jr0MUFXeM6xoZxvTQygHJzmcXzIDUJYUYSi9IimX4bhfEBmxuprzqk4KP2YhgIjUPJrecaghpkf8Diw.png)
1 file for standard output and 1 file for standard error
![home 2 3 > kali > Desktop > Projects > /usr/bin/bash Is l>filel. txt S helloscript.sh (kali@ —'Desktop/projects] $ ./helloscript.sh 2>file2. txt —'Desktop/projects] f nel. txt —'Desktop/projects] file2. txt Is: cannot access No such file or directory (kali@ —'Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d53e8b5f3f253f07e6_CypeqSpRD0Y4-Wt_5-Ra1nef9WfTfedgIPWW8NeeT4jcb2z3oetwdPkommjuXop5xnMioF4wH4UrA60_fmJ3IzuVZbN_Xknxb9OAzhto-zFE7N-ADPtthTFZDUybECDCBtedhGMPuWCZ-GEd3neAdN0.png)
8 Sending Output
![—'Desktop/projects] touch secondscript .sh —'Desktop/projects] L-$ chmod secondscript.sh (kali@ —/Desktop/projects] filel . txt file. txt Iinuxcommands file2 . txt helloscript.sh secondscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d549132b6346fa1a9c_yZnibBB8qBnUl3wOyHR9frC5kZ7tK-0Re8Ppwj4547bUfEUYC6o_kOCOm1ryZYG7VFk993CjZSsW3vQIjLTvpFmhGmsUD2d9OXshcLKM8uJTIOHU_-FiIIV_qV3VZPtHW57XW53dLB91LBhVBnQ_L3M.png)
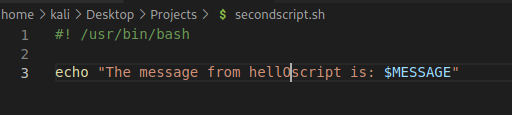
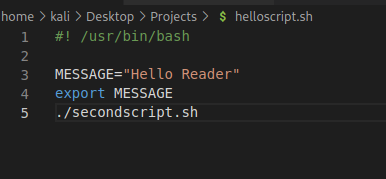
![(kali@ —'Desktop/projects] $ ./helloscript.sh The message from helloscript is: Hello Reader (kali@ —/Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d57c396e4954ef5797_EwiSQ8Ey0lXlnJ5DaEnjytgT_NCbNgquKRWx1oepkT5M6wORzyNOio6q3HN8L3AHC0wmo8b3OuVy9J2EMk7vf2BGvSV6HBQoetx9bDeIBs1VXgoQ0aw-Kr1DRHkOFPhuB1ffs_iZykEBPyXdUj-V44Y.png)
Output from one script is being sent to another
9 Strings Processing
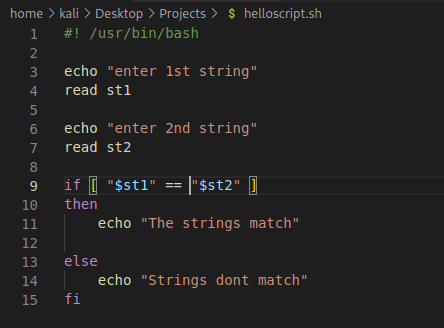
![(kali@ —'Desktop/ projects] $ ./helloscript.sh enter 1st string hello enter 2nd string bye Strings dont match](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d51ee0df783dda332f_ucIYPO4WWzvGqd-fRnMTM5nkHVXSGA1c1DezYNTjacp9-RLAomROp7VFDKc5x3nxfECNyCQXmK093eZevaBR5t1qsSwawz0QW5z_1MOzBkrAW4MrOs5ZWgodRkvEMqGcHgz3EWHCrKh6uCeOcacwkxs.png)
Compares 2 inputs to see if they match
10 Numbers and Arithmetic
To add numbers:

![(kali@ —'Desktop/projects] $ ./helloscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d5672fec437e3ff6b3_CJ3gvvxRRAkrdgjbYHw1CJj-pSZMQx1Pto4HR80Nv4uOQL9qgwDztU4h84WkT1hHHJNGldVdXankNQSfTUA0pIVysSKWVxh7h8PbvObnjylY6UntEQex6Klle3oZGtsjKYBMiFCuZVWWJNzT_TjQYuQ.png)

![(kali@ —'Desktop/projects] $ ./helloscript.sh](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d696977b184c25900a_Y-Xr0WHIyWp9HZzP_g3AUNKg4988LYLicqzT79ihZrvPHaE93ys8a-xvJFWznZIx4y7XDs2wHF7vS1F_ZbjXJj7ou-YwvhXw4KswQCFrfMXfHf88GtA1yEMq84uG3TvnQQ_kAa9MN_o05o8RnXYOykk.png)
Or can use expression
![me > kali > Desktop > Projects > /usr/bin/bash n1=4 helloscript.sh 2 3 4 5 6 7 echo $(expr $nl + (kali@ —'Desktop/projects] $ ./helloscript.sh (kali@ —'Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6c171a922f2d2d59e_lSecAlCRALQ0WF9rsGWW6o9gRP98m2xtdwZggREBs3Yl-dXd6GWbkamOdV3R5tHzwEcazszSV5dP32RRJYxku0NPWl-cy2E9YLnEBE1YGIwp0JtWzW4JXIuXdHFVrEez5rACRMMQXfjAOYmiVo-hTaM.png)
11 Declare Command
Bash does not have a strong type system. Cannot restrict variables, however you can use attributes set by a command. Declare is a bash built in command that allows you to update attributes applied to variables within scope of shell.
Declare -p shows ALL variables
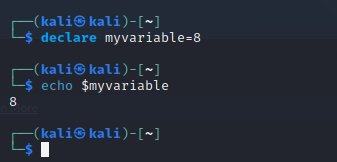
![2 3 4 5 6 7 /usr/bin/bash declare -r pwdfile=/etc/passwd echo "$pwdfile" pwdfile=/etc/abc . txt L$ cd projects (kali@ —'Desktop/ projects] $ ./helloscript.sh /etc/passwd (kali@ —'Desktop/ projects] $ ./helloscript.sh /etc/passwd ./helloscript.sh: (kali@ line 7: pwdfile: readonly variable —'Desktop/ projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6613ef284abe5d0fa_Hi0gTMsEE-4sN155hEWA6YbbkNqg-15u2y7fpU8bxRpROrfw9_UE0W23FKBGWt4H3ha9iGpBJ7FXyl7f3ROynbf2UPlbGHWdrmAbO0V7wJv3-OpNBiLpMF18_U_om6X10KMU-7A6WUwWa4ex54rmzgQ.png)
Can see that it is trying to print out twice. This is because pwd file is being declared as a read variable
12 Arrays
![/usr/bin/bash 2 3 4 5 • 'Honda echo 'BMW' 'Toyota "${car (kali@ —'Desktop/projects] $ ./helloscript.sh BMW Toyota Honda (kali@ —/Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d66433bee0913c5acd_bNVHReM6LZL9WXNig1t-a0qIrE7gy_Rb2pfhYSsvPJRdB7H8JKBPvRh6rMgyvXZb3wqL-vJC8JAw6nnI6eFdArJ8uL4-SDjHYDuFgKLHuCOETgbAfhHOm4VfkEYVqoEFRTZbkDl13N6vMUnTH6A7dOc.png)
Prints out all of the arrays
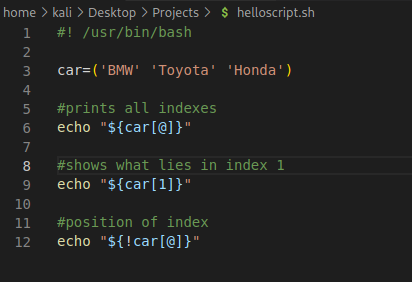
![(kali@ —'Desktop/projects] $ ./helloscript.sh BMW Toyota Honda Toyota 012](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6dec80530a2100f23_KRz2wRbRU4mW0VIIflRLKHqiLEYN2swPQK5vzAYZ1cNYUCzz1D59W6TlfTiylnbPXtuKWmAZLVY8qPwNOcHY4e-Ixf3F2C8VTn9FnuZkwn_Xa9NPIvmArPx01yx91nsIeLDHoV17IOTPEiEnh2rb13A.png)
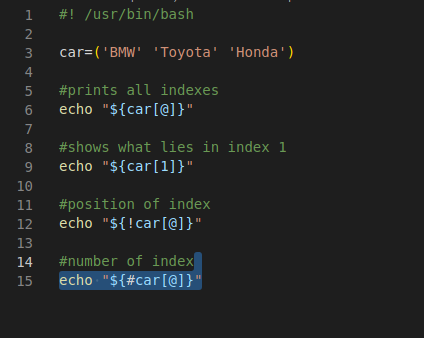
13 Functions
In order to make functions work, they need to be called
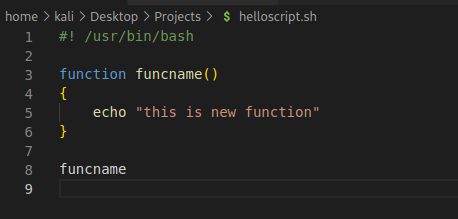
![(kali@ —'Desktop/projects] $ ./helloscript.sh this is new function (kali@ —/Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6f86a44478c8e917d_prrxKC9UiP3qjPPw4T9nDA53jvVfdhzONA-vVb1LzPEagVpAQvlC_jxK4td_crHnhRifKsGDIuFLt4XCt0tziqOFRZxTc6P_dCiywoKKHfCHqfKfrQ_AtsxGsNS5-itwQ0v2lnGgVyrBOnrFRllYgJk.png)
14 Files and Directories
Learn how to create directories/check if current directories exist or not, how to append text from file and how to read line by line

![(kali@ —/Desktop/projects] filel . txt file2 . txt file . txt helloscript.sh (kali@ —/Desktop/projects] Iinuxcommands secondscript. sh testfolder](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d65e43cb8f14d6b72d_IUoq1qAgXA7GBfqkSlb0dcTHZAmAcOoGpvwAXhd1BzhJFgQ52SO6QL2BGOIFa0-hH9GwdDdnFmHgnCa4vbXYOZUoHzwrdkHokLK9ljKRpF5juLi_ZsMUomzXCA56Qv8Kc0h27rZoQEiNQHX50E-TLSA.png)
![home > kali > Desktop > Projects > /usr/bin/bash S helloscript.sh 2 3 4 5 6 7 8 9 10 12 13 echo "Enter Directory name to check" read direct if [ -d "$direct" ] t hen echo else echo fi "directory exists" "directory doesnt exist"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6566c6e196139e371__yL3tfSlvYC-tv162N-KrUQ4CNnXa6iTWsCGMtWrt8YLXfCjOyOgmJ05mVPZ0Gdf6a-xyPfGi0YiTz3em4rdtNQT4TkYyXTNqYrlNy_AQy3pcSiptIhQ8FwY8TmmLfhUKYmD_sek6LHAbu6ujqLxjCY.png)
![filel . txt file2 . txt file . txt helloscript.sh (kali@ —'Desktop/projects] $ ./helloscript.sh Enter Directory name to check testfolder directory exists (kali@ —'Desktop/projects] $ ./helloscript.sh Enter Directory name to check jkl directory doesnt exist Iinuxcommands secondscript. sh testfolder](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d641d4f4f0b8778b4c_6njbffZ9JDV_Q7KOXvZUjtKSLc2_pa8Tzs-yJ52vkleemXvwZWGMTHh6xMFijMk9IijJwlkC_F2M47-U3I-H4iUxqc_JHpQKMstan8iPKPnHos4Fhfw6M275pVhccC_PU7-RRfNqUa04VWeY5oz9MN4.png)
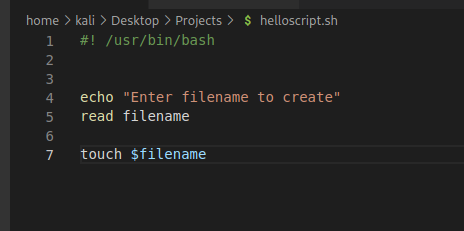
Creating a filename
![(kali@ —'Desktop/projects] $ ./helloscript.sh Enter filename to create testedfile (kali@ —/Desktop/projects] filel . txt file. txt Iinuxcommands file2 . txt helloscript.sh secondscript.sh testedfile testfolder](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6d5014d2289e241be_dbF0i--d1A4PFW87rNXKsxbA6xcxzInQb-279uWxwZr6hRLoCQzGFFVMrP6S3RIYvHs5KDgpYvqZ3rGFz73VQWc-ck5BC-AV2s04IxguCCTQOb5Rsowpdjnp1pw5kQFulrc2HGD_OLPyooHXblXAWuA.png)
![S helloscript.shX $ secondscript.sh home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 /usr/bin/bash echo "Enter filename to create" read filename touch $filename echo "enter filename to check" read filename if [ [ -f t hen echo else echo fi "$filename" ] ] "$filename exists" "$filename doesnt exist"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d6da6e6421bba8ce31_cNX7W02FIT86X9OfmFOf3enMSu2WKNgGr3TCPMGlQaTsySXXsUur4nolxA_INmJzg8hxTE4iqpj809lzsmaAcsx-44nD8Es72fV3qIL6qHuIKi3y8nYAr3l6w3XHH5PuMHjio_TrGd172lOOFL8VWtI.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh Enter filename to create jelanitest enter filename to check jelanitest jelanitest exists](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d65e43cb8f14d6b746_unpsRh2L9LevpmYNvqmUG2ruyvbOzZQUwWC7SyBe7_Gf6zedWkm_xH-O_F_iZ4fRvzmDePZyobJ366i99a7aoQIrc6DXC4lA2OLRejvq_uGoePkKkrX_o92KbD5pJblD1z_jOKO8QGae2bpj4UGxvU8.png)
15 Curl in scripts

16 Professional menus
Select loops and how to work with it
![S helloscript.shX $ secondscript.sh home > kali > Desktop > Projects > S helloscript.sh /usr/bin/bash Rover Toyota ./helloscript .sh: line 6: syntax error: (kali@ —'Desktop/projects] $ ./helloscript.sh 2) Mercedes 3) Tesla 4) Rover 5) Toyota You have selected Tesla You have selected Toyota You have selected BMW You have selected unexpected end of fi 2 3 4 5 6 select car in do echo "You done Mercedes Tesla have selected $car"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d66433bee0913c5ba5_Qj_zjaNWGFcVDxmxOAD6mkJhFr7cAufmlQI7SZPsEPIMaYiDb1udMikQGJST0YGoOYrEVPYSq4hRVfhS-kYoDiaXC9lSUpGlUEzSMpF6cfatoYGfJhkuoFoqSFD0HIzYUfO7QqL_BUx3nKAv2yAmZqY.png)
Needs a check statement.
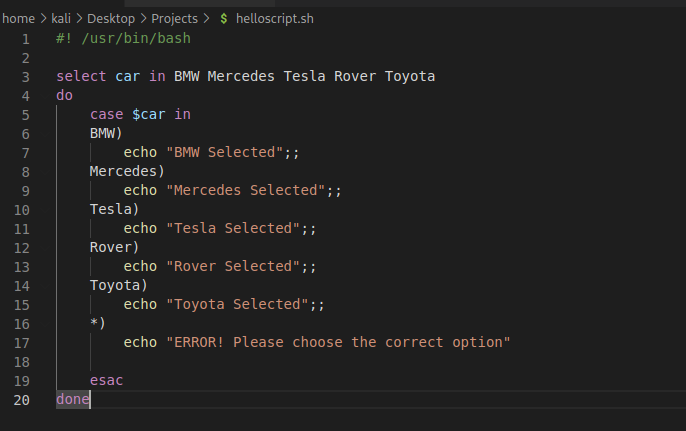
![(kali@ —'Desktop/projects] $ ./helloscript.sh 1) BMW 2) Mercedes 3) Tesla 4) Rover 5) Toyota 89 ERROR! Please choose the correct option](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d79226cae589a6de9f_dESjQHIroPusTAX_C3gLpAhZltjbz9kAewr2zInL8wfFInB2A8t_tRviAjHEmiAWpZ-R4R1cnNV8QKZCaF3ZL3yoSu7bTnC1vsrM0K3vT3vuKxnqA9uI33GpPPjTBWFow9qcPRCAJQx8ycf7X3L_7-k.png)
17 Wait for filesystem events with inotify
Inotify is a linux kernel subsystem that acts to extend file systems. Whatever changes are made inotify will monitor the files and directories
![(kali@ —'Desktop/projects] $ ./helloscript.sh Setting up watches. Couldn't watch /temp/newfolder: No such file or directory (kali@ —/Desktop/projects]](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d7cb78b6f1ed760394_jpUK21_AFD3PlpdQIvGy8drY6SMbUlQtEgqd87BbkThbsl1a5iOvbz0dKh2LAQ6ThM1fgq4aShl8eTd2mGzYa9_nUCafjWS4tyfPjFgtbGlIrIw0DNcNzvstXR0k-GPmZ3RuYgdEC60A7m0ErcZkuYc.png)
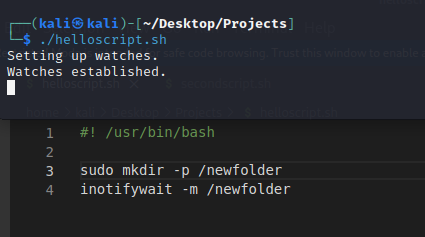
18 Introduction to grep

![S helloscript.sh X filegrep.txt $ secondscript.sh home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 8 9 1€ 11 12 13 14 15 /usr/bin/bash echo "Please enter the file to search text from: " read filename if [ [ -f t hen echo read g rep else echo fi $filename ] ] "enter text to search" grepvar -i $grepvar $filename "file doesnt exist"](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d777e47df09cb1cdc4_APUAbtid3wGwH8BLaawd_nfWBRzentknp2pGrdgervS6jXh8bHpJNbeCwCXxWnrh7wIaNtZ9cEJccc7mcgKxaPO1KjLp6hZqyLLcAc20JcehOMeD3cadXUzhqqWUL8diF-We2Wo8X7SAPMWZ-FKpo_4.png)
19 Introduction to awk
Awk - scripting language used to manipulate data and generate reports
![home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 8 9 10 11 12 /usr/bin/bash echo "Please enter the file to print from awk" read filename if [ [ -f $filename J] t hen awk else echo /windows/ {print}' "file doesnt exist" $filename](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d722d5eaa2ef6c6779_zgWbw3iWt71xjyE1CQdmCAVn-xQtATMhc8IVh2du7LLsH-tEB-2IN3e7cN_rs3mGvcQZ3XDY7h-BCaBv_bwj6dEFCvdZ1aOkr-yZ6Hg8bNYHZfjGMsI8Tdt41LPgulEEew_Xr_5fceq8VBbw8DV5SLs.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh Please enter the file to print from awk filegrep . txt This is windows](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d7ebe0056d7d491db0_bdq_9IJTbMEJ0iG3L106jdqqTlmFxfDrVFeLf2INubehyw_JRX9U2-cjB5oqtAZ-bw4XgZcppWtWnX2Lf644gY10t9JAFcDl0AAQLBTQ8QqkdE-VlH-ie6C7woeEOpvYQHGvM9UNmivAC5ibpNJ5-Ro.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh Please enter the file to print from awk filegrep . txt](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d7613ef284abe5d1f4_HIr9qslLTS4fmZEnj-yUKdGlijKcuYvzO9mapN5MMRu-9OzkqleRV2iGjj8SlMEsozBRMgckwq0cDmayL-eJe7LXnKYKGcxSz7r2zB3nEtkXLquqFq7bQF08oETxHm-qePlKTp0WLvupV42_XUs0p0Y.png)
![home > kali > Desktop > Projects > S helloscript.sh 2 3 4 5 6 7 8 9 10 11 12 /usr/bin/bash echo "Please enter the file to print from awk" read filename if [ [ -f $filename J] t hen awk else echo fi ' /windows/ {print $21} "file doesnt exist" $filename](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d739f6a9c478a2b055_SqNOyg9pTynnRLucPLfXif3Cc8QbasSFGVu_7wyEB0_1fp80Ky1ikB-LqHVarTxZSHCYtiiDzEOIsNR16ltT_UUOOZv0ZkIqVD55oPW8eH6a5_qGQcbCIfcj_9Qv5M9zMaxBeVsc9AL4TjX5l3wdLZA.png)
20 Introduction to sed
Sed stands for stream editor and can be used to manipulate text files
![(kali@ —'Desktop/projects] $ ./helloscript.sh Please enter the filename to substitute using sed filegrep . txt Thls is Linux Thls is windows Thls is mac](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d75e43cb8f14d6b85a_vbZbPiUlcEGK6RC1cMNt8Nae_rpG-XosQroE52XVw0dY-iklO_Acezfc7TFindpkbVIRopb0Dg69tDNDVGh-e23wVlm7zSD2ApTxo8GNYJzKuoXvaXYREVjtAlCvTeSyCDXLtybGJ4UhwvYZQzvXcb0.png)
![(kali@ —'Desktop/projects] $ ./helloscript.sh Please enter the filename to substitute using sed filegrep . txt This Is Linux Thls Is wlndows Thls Is mac](https://cdn.prod.website-files.com/643b49e21052bb7e68da0606/6499e8d73962f3dd04b1bf07_ork0sD3MZa1wGSSiiclyZ0V7fWDWEK7n15NnXgwwfGbCY63xmsQXSCegnjy-ptLAT-LGx7Laoc1bJkNBF3OpiNvHAtDtzqcYZkeh4w8H8_jF5kEE1dnKDkpxihqtI8pFNT887-FTV1Idqd4TxnGnyNk.png)
21 - Debugging Bash Scripts
Bash offers an extensive debugging facility so you know if it doesn’t go according to plan. The error includes the line and where the error is
Bash -x shows you the code that is being executed.